Table Of Content
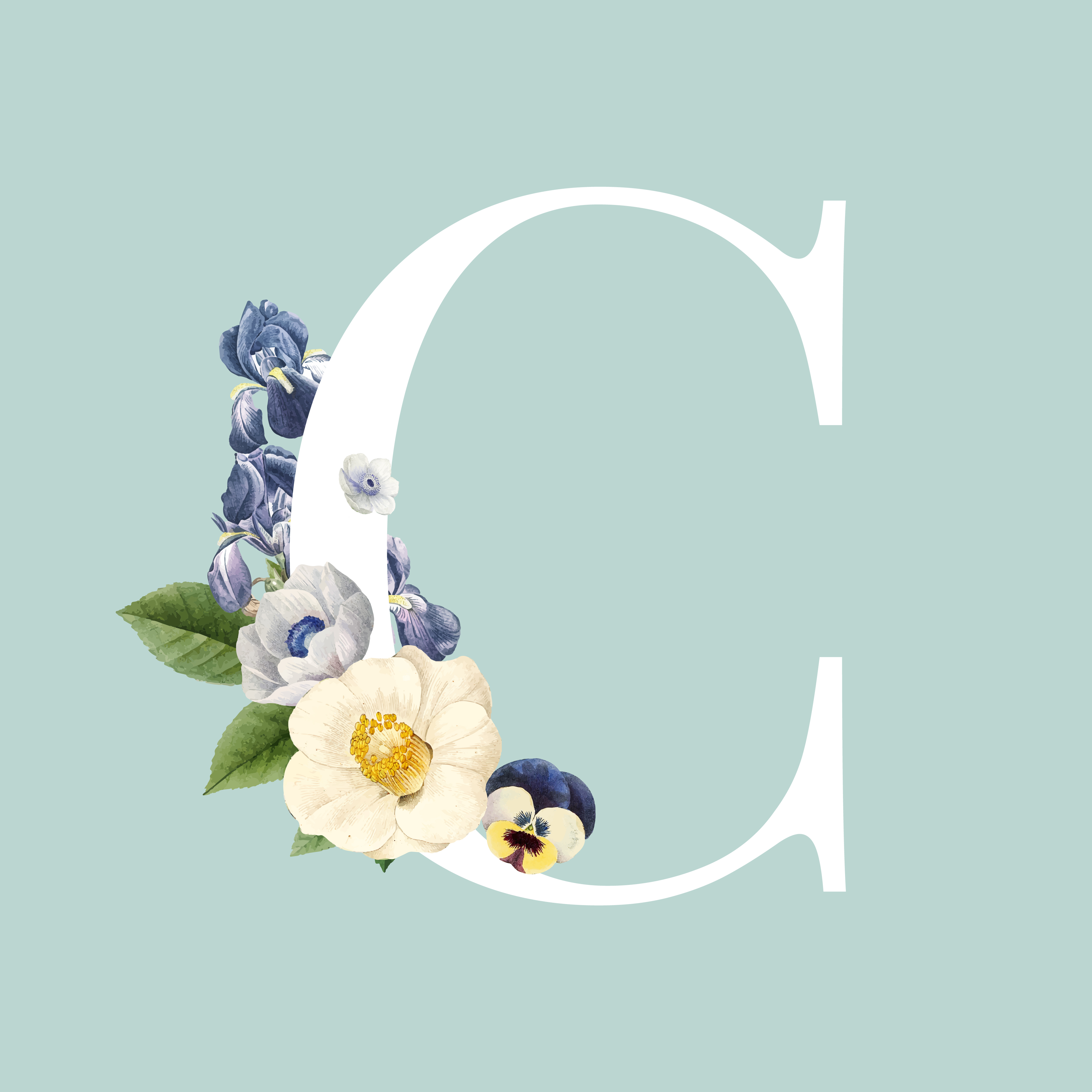
Objects with automatic storage are local to the block in which they were declared and are discarded when the block is exited. Additionally, objects declared with the register storage class may be given higher priority by the compiler for access to registers; although the compiler may choose not to actually store any of them in a register. Objects with this storage class may not be used with the address-of (&) unary operator. In this way, the same object can be accessed by a function across multiple calls.
File handling and streams
Objects with allocated storage duration are created and destroyed explicitly with malloc, free, and related functions. Most of them also express highly similar syntax to C, and they tend to combine the recognizable expression and statement syntax of C with underlying type systems, data models, and semantics that can be radically different. C (pronounced /ˈsiː/ – like the letter c)[6] is a general-purpose computer programming language. It was created in the 1970s by Dennis Ritchie, and remains very widely used and influential.
K&R C
It includes a number of features not available in normal C, such as fixed-point arithmetic, named address spaces, and basic I/O hardware addressing. Historically, embedded C programming requires nonstandard extensions to the C language in order to support exotic features such as fixed-point arithmetic, multiple distinct memory banks, and basic I/O operations. This concludes our first part, and we merely scratched the surface of possibilities we have with pointers, which just shows how complex the seemingly simple concept of “it’s just a memory address” can really be. Next time, we continue with pointer arithmetic and some more complex pointer arrangements.
POSIX standard library
The standard macro __STDC_VERSION__ is defined as L to indicate that C11 support is available. The original C language provided no built-in functions such as I/O operations, unlike traditional languages such as COBOL and Fortran.[citation needed] Over time, user communities of C shared ideas and implementations of what is now called C standard libraries. Many of these ideas were incorporated eventually into the definition of the standardized C language. On Unix-like systems, the authoritative documentation of the API is provided in the form of man pages. On most systems, man pages on standard library functions are in section 3; section 7 may contain some more generic pages on underlying concepts (e.g. man 7 math_error in Linux).
Rationale for use in systems programming
Programs written for hosted implementations are required to define a special function called main, which is the first function called when a program begins executing. The result is a "pointer to int" variable (a) that points to the first of n contiguous int objects; due to array–pointer equivalence this can be used in place of an actual array name, as shown in the last line. The advantage in using this dynamic allocation is that the amount of memory that is allocated to it can be limited to what is actually needed at run time, and this can be changed as needed (using the standard library function realloc). The enumerated type in C, specified with the enum keyword, and often just called an "enum" (usually pronounced /ˈiːnʌm/ EE-num or /ˈiːnuːm/ EE-noom), is a type designed to represent values across a series of named constants. Each enum type itself is compatible with char or a signed or unsigned integer type, but each implementation defines its own rules for choosing a type. The syntax of the C programming language is the set of rules governing writing of software in C.
The type qualifier volatile indicates to an optimizing compiler that it may not remove apparently redundant reads or writes, as the value may change even if it was not modified by any expression or statement, or multiple writes may be necessary, such as for memory-mapped I/O. The char type is distinct from both signed char and unsigned char, but is guaranteed to have the same representation as one of them. The _Bool and long long types are standardized since 1999, and may not be supported by older C compilers. Type _Bool is usually accessed via the typedef name bool defined by the standard header stdbool.h.
Assembling Data with Pointers
At Version 4 Unix, released in November 1973, the Unix kernel was extensively re-implemented in C.[8] By this time, the C language had acquired some powerful features such as struct types. Semicolons terminate statements, while curly braces are used to group statements into blocks. The most important requirement to succeed with pointers and avoid segmentation faults is that they always have a large enough place inside memory that they can actually access. Again, on a system without MMU, any location in the memory is technically such a place, but it’s a lot stricter with memory protection, and we also need to avoid pointers that don’t point to anywhere specific.
Obituary for Steven C. Calvelage - Snyder & Hollenbaugh Funeral & Cremation Services
Obituary for Steven C. Calvelage.
Posted: Thu, 25 Apr 2024 19:50:21 GMT [source]
The for statement has separate initialization, testing, and reinitialization expressions, any or all of which can be omitted. Break is used to leave the innermost enclosing loop statement and continue is used to skip to its reinitialisation. There is also a non-structured goto statement which branches directly to the designated label within the function. Switch selects a case to be executed based on the value of an integer expression.
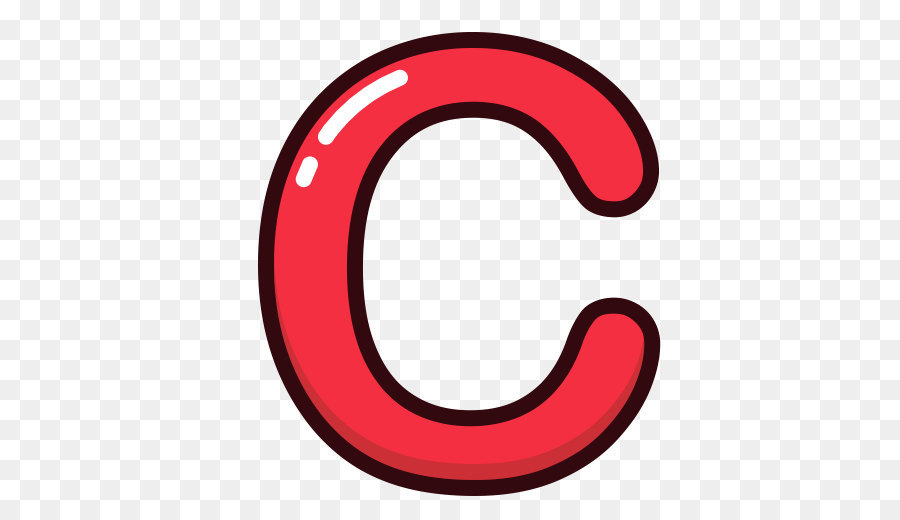
If two pointers to the same function are derived in two different translation units in the program, these two pointers must compare equal; that is, the address comes by resolving the name of the function, which has external (program-wide) linkage. Yes, your microcontroller registers are in fact pointers to a hard-coded memory address. If you take a look at the Register summary section in the ATmega’s data sheet, you will find those two registers are mapped indeed to addresses 0x24 and 0x25 respectively. While individual strings are arrays of contiguous characters, there is no guarantee that the strings are stored as a contiguous group.
Heap memory allocation has to be synchronized with its actual usage in any program to be reused as much as possible. For example, if the only pointer to a heap memory allocation goes out of scope or has its value overwritten before it is deallocated explicitly, then that memory cannot be recovered for later reuse and is essentially lost to the program, a phenomenon known as a memory leak. Conversely, it is possible for memory to be freed, but is referenced subsequently, leading to unpredictable results.
The first two definitions are equivalent (and both are compatible with C++). It is probably up to individual preference which one is used (the current C standard contains two examples of main() and two of main(void), but the draft C++ standard uses main()). The return value of main (which should be int) serves as termination status returned to the host environment. C's string-literal syntax has been very influential, and has made its way into many other languages, such as C++, Objective-C, Perl, Python, PHP, Java, JavaScript, C#, and Ruby. Nowadays, almost all new languages adopt or build upon C-style string syntax. Again, reading from left to right, this accesses the 5th row, and the 4th element in that row.
If there are no parameters, the may be left empty or optionally be specified with the single word void. Because of the language's grammar, a scalar initializer may be enclosed in any number of curly brace pairs. Most compilers issue a warning if there is more than one such pair, though. String literals may not contain embedded newlines; this proscription somewhat simplifies parsing of the language. To include a newline in a string, the backslash escape \n may be used, as below. The benefit to using the second example is that the numeric limit of the first example isn't required, which means that the pointer-to-array could be of any size and the second example can execute without any modifications.
White House Correspondents Association Dinner Arrivals - C-SPAN
White House Correspondents Association Dinner Arrivals.
Posted: Sat, 27 Apr 2024 21:02:49 GMT [source]
With while, the test, including all side effects from , occurs before each iteration (execution of ); with do, the test occurs after each iteration. Thus, a do statement always executes its sub-statement at least once, whereas while may not execute the sub-statement at all. Unnamed fields consisting of just a colon followed by a number of bits are also allowed; these indicate padding. Future statements can then use the specifier s_type (instead of the expanded struct ... specifier) to refer to the structure.
Though logically the last subscript in an array of 10 elements would be 9, subscripts 10, 11, and so forth could accidentally be specified, with undefined results. This specifies most basically the storage duration, which may be static (default for global), automatic (default for local), or dynamic (allocated), together with other features (linkage and register hint). During the late 1970s and 1980s, versions of C were implemented for a wide variety of mainframe computers, minicomputers, and microcomputers, including the IBM PC, as its popularity began to increase significantly. According to the C standard the macro __STDC_HOSTED__ shall be defined to 1 if the implementation is hosted. An implementation can also be freestanding which means that these headers will not be present. If an implementation is freestanding, it shall define __STDC_HOSTED__ to 0.
Array types in C are traditionally of a fixed, static size specified at compile time. However, it is also possible to allocate a block of memory (of arbitrary size) at run-time, using the standard library's malloc function, and treat it as an array. Most of the recently reserved words begin with an underscore followed by a capital letter, because identifiers of that form were previously reserved by the C standard for use only by implementations. Since existing program source code should not have been using these identifiers, it would not be affected when C implementations started supporting these extensions to the programming language. Some standard headers do define more convenient synonyms for underscored identifiers.
No comments:
Post a Comment